Welcome to Tonder Developer Docs!
Tonder is an all-in-one infrastructure that streamlines payment processing, supports various payment methods, and offers customizable integrations to enhance payment success, prevent fraud, and optimize customer experience.
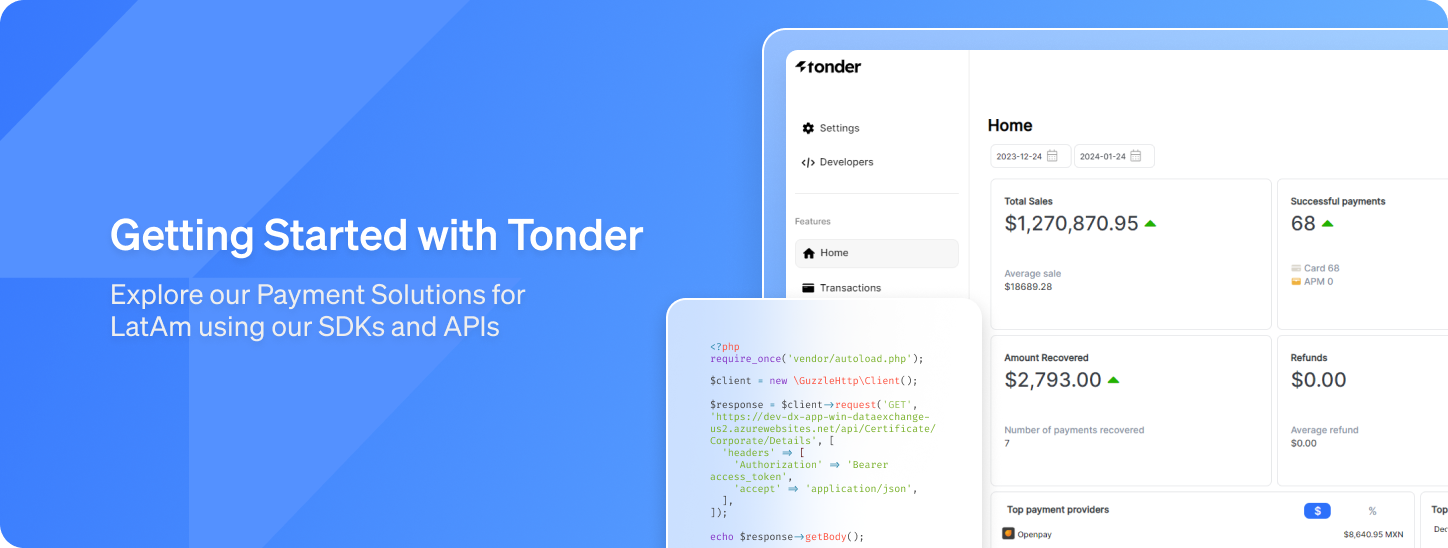
Benefits
Tonder offers a simplified and secure way of making online payments. Tonder empowers your businesses to effectively manage:
- Diverse payment methods
- Robust fraud prevention measures
- Optimal processing costs
To take advantage of Tonder solution you use a single integration that allows you to accept all payment methods and manage fraud effectively. This unified approach enhances both the payment process and overall customer experiences. Our solution effectively tackles common challenges, including low approval rates, costly development processes, and insufficient fraud management.
Guides
Get Started
Learn how Tonder works and get a broader knowledge about payments.
Core Concepts
Online Payments
Tonder Online Payments offers merchants a streamlined and secure solution for conducting online transactions.
Revenue Optimization
Tonder’s Revenue Optimization layer streamlines and optimizes the integration and management of various payment services and processes in one single platform.
Integrate Tonder
Integrate Tonder into your applications using SDKs and plugins.
Dashboard
Tonder’s dashboard is where you can manage all of your transactions, track performance and much more.
API Reference
Check and test the API endpoints used to integrate with Tonder.